C#のクラスメンバーについてさらに書いていきます。
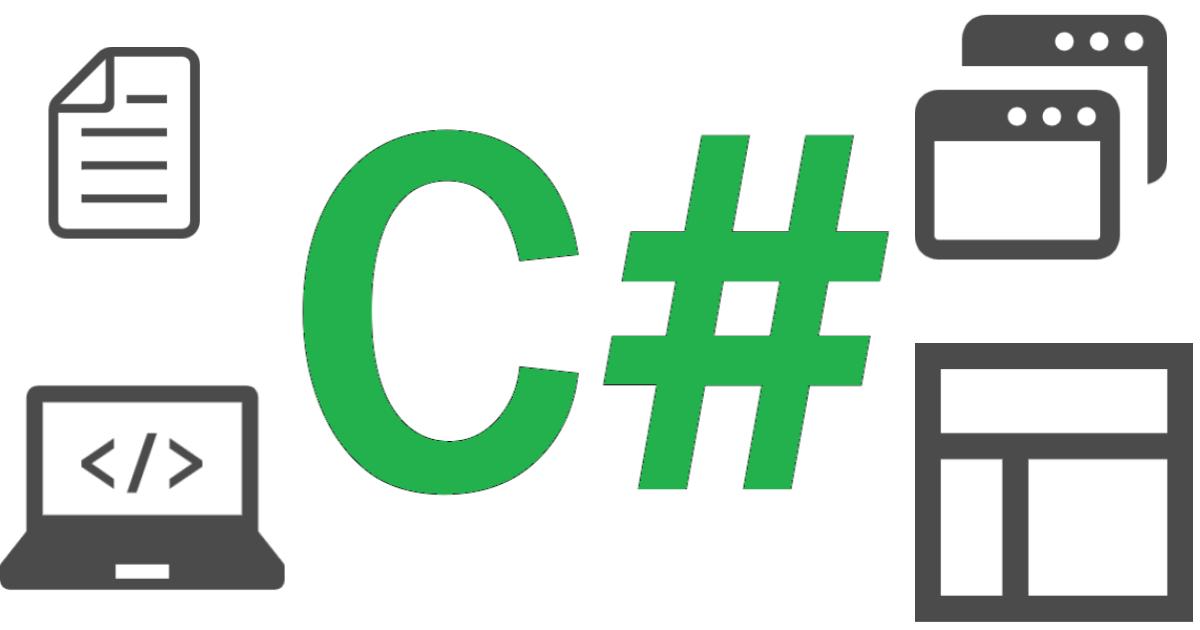
[C# 入門] クラス(class)について
クラス(class)とは クラスは値や変数、メソッドなどをひとまとまりにしたものです。とりあえずこれだけ覚えておきましょう。 もう少しちゃんと書くと、クラスはユーザー定義型と言って、開発者が変数やメソッドを自由に組み合わせて1つの新しいデー
上の記事では変数(フィールド)、メソッドをクラスのメンバーとして説明しました。
この記事ではさらにメンバーを説明します。
ざっくりとメンバーの種類の一覧です。
- フィールド
- プロパティ
- メソッド
- コンストラクター
- ファイナライザー
- イベント
- インデクサー
今回はよく使われるコンストラクターです。
コンストラクターとは
コンストラクターはクラスがオブジェクト化(インスタンス化)される際に呼び出される特殊なメソッドです。
クラス名と同じ名前のメソッドがコンストラクターになります。
戻り値の型は書きません。
コンストラクターの使い方
わかりにくいと思いますが、クラスをnewで作成するときに1回だけ呼ばれるメソッドと覚えてください。
これを利用することで、クラス内の変数に初期値を設定することができます。
public class ClassSample
{
// メンバー変数
public int x;
public int y;
// コンストラクター(クラス名と同じ名前のメソッド)
public ClassSample() {
// メンバー変数の初期値を設定
this.x = 10;
this.y = 20;
}
}
thisというキーワードはそのクラスのインスタンスのことです。
ここでの「this.x」はClassSambleのメンバー変数xを指しています。
クラスをnewを使ってインスタンスを作成したときにコンストラクターが呼び出されます。
class Program
{
static void Main(string[] args)
{
ClassSample c = new ClassSample(); // ここでコンストラクターが呼ばれる
}
}
パラメータをもつコンストラクター
コンストラクターに引数(パラメーター)を指定できます。
public class ClassSample
{
// メンバー変数
public int x;
public int y;
// コンストラクター
public ClassSample(int x, int y) {
// メンバー変数の初期値を設定
this.x = x;
this.y = y;
}
}
class Program
{
static void Main(string[] args)
{
// ↓ここでコンストラクターが呼ばれる
ClassSample c = new ClassSample(10, 20);
}
}
new ClassSample(10, 20); newを使う際のクラス名の後ろにパラメータを指定します。
パラメータあり、なし両方でコンストラクターを書いて状況で使い分けたりします。
public class ClassSample
{
// メンバー変数
public int x;
public int y;
// コンストラクター1
public ClassSample() {
this.x = 10;
this.y = 20;
}
// コンストラクター2
public ClassSample(int x) {
this.x = x;
}
// コンストラクター3
public ClassSample(int x, int y) {
this.x = x;
this.y = y;
}
}
class Program
{
static void Main(string[] args)
{
ClassSample c1 = new ClassSample(); //コンストラクタ1が呼ばれる
ClassSample c2 = new ClassSample(10); //コンストラクタ2が呼ばれる
ClassSample c3 = new ClassSample(10, 20); //コンストラクタ3が呼ばれる
}
}
ちなみに、メソッドは同じ名前でパラメータの数が違う場合に複数宣言することができます。オーバーロードと言います。
まとめ
今回のまとめ
- コンストラクターはクラスメンバーの種類の1つ
- クラス名と同じ名前の特殊なメソッド
- newキーワードでクラスのインスタンスが作成されるとき一度だけ呼ばれる
- 1つのクラスにオーバーロードで複数のコンストラクターを持つことができる
クラス関連の記事はこちら
リンク
リンク
C# 記事まとめページに戻る(他のサンプルコードもこちら)
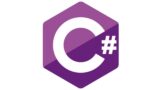
C# プログラミング講座
C#についての記事まとめページです。開発環境VisualStudioのインストール方法や使い方、プログラミングの基礎知識についてや用語説明の記事一覧になっています。講座の記事にはすぐに実行できるようにサンプルコードを載せています。
コメント